A common question from users transitioning from task-centric engines (like Camunda) to Imixs-Workflow, is whether Imixs-Workflow supports Service Tasks—a common BPMN element used in workflow engines like Camunda. Let’s see how this works in Imixs-Workflow
First of all – you can add a service Task into your model. But this will not execute any code.
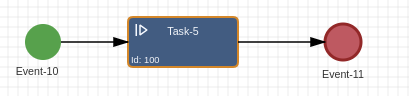
The answer to this lies in understanding Imixs’s event-driven architecture. Unlike BPMN engines that rely on embedded code in Service Tasks, Imixs executes custom logic through events by two core mechanisms:

1. Plugins: Reusable Workflow Logic
Plugins are Java classes triggered at the processing phases of the workflow lifecycle. They act like “global interceptors” for business logic.
Key Traits:
- Extend
org.imixs.workflow.Plugin
orAbstractPlugin
. - Running in each processing cycle
- Modify workflow documents during processing.
A Plugin can be added into the Model Workflow definition and will be executed automatically in each processing cycle.
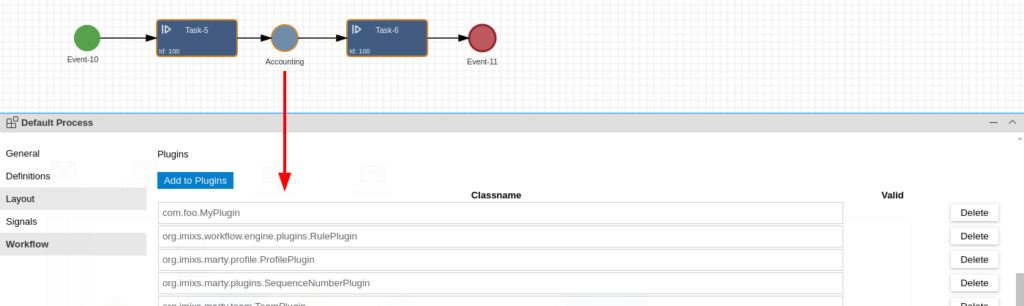
Example: Automatically calculate a discount in an order approval workflow:
public class DiscountPlugin extends AbstractPlugin {
@Override
public ItemCollection run(ItemCollection document, ItemCollection event)
throws PluginException {
if ("approve".equals(event.getItemValueString("name"))) {
double total = document.getItemValueDouble("total");
document.replaceItemValue("discount", total * 0.1); // 10% discount
}
return document;
}
}
(See Plugin API Docs for details.)
2. Adapters: Event-Driven Actions
Adapters (CDI observers) react to workflow events, similar to microservices listening to Kafka topics. They’re ideal for side effects (e.g., sending emails, logging).
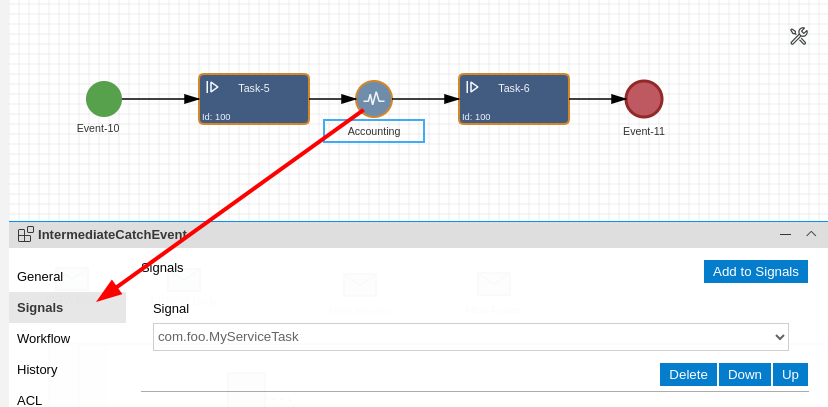
Key Traits:
- Support CDI / EJB / Transactional
- Running on a specific Event
- Decoupled from the core workflow (run asynchronously if needed).
Example:
public class DemoAdapter implements SignalAdapter {
// inject services...
@Inject
ModelService modelService;
...
@Override
public ItemCollection execute(ItemCollection document, ItemCollection event) throws AdapterException {
if ("approve".equals(event.getItemValueString("name"))) {
double total = document.getItemValueDouble("total");
document.replaceItemValue("discount", total * 0.1); // 10% discount
}
return document;
}
}
Why This Approach?
- Separation of Concerns: Business logic lives outside BPMN files.
- Flexibility: Plugins/Adapters can be shared, tested, and versioned independently.
- Scalability: Events integrate with Java EE/CDI, Kubernetes, or serverless.
Migration Tip for Camunda Users
Instead of embedding code in a Service Task:
- For document transformations: Use a
Plugin
. - For side effects (APIs, notifications): Use an
Adapter
.
Explore Further:
Questions? Discuss in the Imixs Forum.